mob 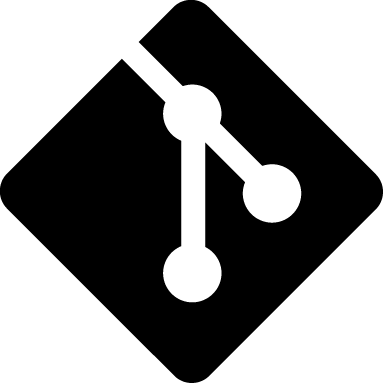
CLICKDELAY HANDLING SYSTEM How this works is mobs can never do actions until their next_action is at or below world.time, but things can specify extra cooldown to check for either from the time of last_action or from the end of next_action.
Clickdelay should always be checked via [CheckActionCooldown()], never manually!
Vars | |
LAssailant | The last mob/living/carbon to push/drag/grab this mob (mostly used by slimes friend recognition) |
---|---|
ability_actions | ability = action button instance. |
ability_properties | ability = list(data). see __DEFINES/mobs/innate_abilities.dm |
action_cooldown_adjust | Simple modification variable added to amount on adjust and on checking time since last action using [CheckActionCooldown()]. This should only be manually modified via addition. |
action_cooldown_mod | Simple modification variable multiplied to next action modifier on adjust and on checking time since last action using [CheckActionCooldown()]. This should only be manually modified using multipliers. |
actionspeed_mod_immunities | List of action speed modifiers ignored by this mob. List -> List (id) -> List (sources) |
actionspeed_modification | List of action speed modifiers applying to this mob |
cached_multiplicative_actions_slowdown | The calculated mob action speed slowdown based on the modifiers list |
cached_multiplicative_slowdown | The calculated mob speed slowdown based on the modifiers list |
deathsound | The sound made on death |
do_afters | For storing what do_after's someone has, in case we want to restrict them to only one of a certain do_after at a time |
examine_cursor_icon | Cursor icon used when holding shift over things |
focus | What receives our keyboard input. src by default. |
fullscreens | Fullscreen objects |
innate_abilities | Ability system based on action buttons. Can be ported to base /mob or /mob/living later if needed, easily - the procs are currently on living/carbon/human/innate_abilities.dm datum traits-style lazylist of abilities |
is_shifted | Whether the mob is pixel shifted or not |
last_action | Generic clickdelay variable. Marks down the last world.time we did something that should cause or impact generic clickdelay. This should be directly set or set using [DelayNextAction()]. This should only be checked using [CheckActionCooldown()]. |
last_action_immediate | The difference between the above and this is this is set immediately before even the pre-attack begins to ensure clickdelay is respected. Then, it is flushed or discarded using [FlushLastAttack()] or [DiscardLastAttack()] respectively. |
mob_transforming | Whether or not the mob is currently being transformed into another mob or into another state of being. This will prevent it from moving or doing realistically anything. Don't you DARE use this for a cheap way to ensure someone is stunned in your code. |
mock_client | A mock client, provided by tests and friends |
movespeed_mod_immunities | List of movement speed modifiers ignored by this mob. List -> List (id) -> List (sources) |
movespeed_modification | List of movement speed modifiers applying to this mob |
next_action | Generic clickdelay variable. Next world.time we should be able to do something that respects generic clickdelay. This should be set using [DelayNextAction()] This should only be checked using [CheckActionCooldown()]. |
next_action_immediate | Ditto |
next_resist | Minimum world time for another resist. This should only be checked using [CheckResistCooldown()]. |
passthroughable | Takes the four cardinal direction defines. Any atoms moving into this atom's tile will be allowed to from the added directions. |
resist_cooldown | How long we should wait before allowing another resist. This should only be manually modified using multipliers. |
shifting | If we are in the shifting setting. |
sound_environment_override | Override for sound_environments. If this is set the user will always hear a specific type of reverb (Instead of the area defined reverb) |
tgui_open_uis | global |
typing_indicator_current | Current state of our typing indicator. Used for cut overlay, DO NOT RUNTIME ASSIGN OTHER THAN FROM SHOW/CLEAR. Used to absolutely ensure we do not get stuck overlays. |
typing_indicator_enabled | ////TYPING INDICATORS/////// Set to true if we want to show typing indicators. |
typing_indicator_state | Default icon_state of our typing indicator. Currently only supports paths (because anything else is, as of time of typing this, unnecesary. |
typing_indicator_timerid | The timer that will remove our indicator for early aborts (like when an user finishes their message) |
unarmed_attack_speed | Default clickdelay for an UnarmedAttack() that successfully passes. Respects action_cooldown_mod. |
Procs | |
CheckActionCooldown | Checks if we can do another action. Returns TRUE if we can and FALSE if we cannot. |
CheckResistCooldown | Checks if we can resist again. |
CommonClickOn | Common mob click code |
DelayNextAction | Applies a delay to next_action before we can do our next action. |
DiscardCurrentAction | Discards last_action and next_action |
Dizzy | Set the dizzyness of a mob to a passed in amount |
EstimatedNextActionTime | Get estimated time of next attack. |
FlushCurrentAction | Flushes last_action and next_action |
GetActionCooldownAdjust | Gets action_cooldown_adjust |
GetActionCooldownMod | Gets action_cooldown_mod. |
Jitter | Set the jitter of a mob |
MarkResistTime | Mark the last resist as now. |
SetNextAction | Sets our next action to. The difference is DelayNextAction cannot reduce next_action under any circumstances while this can. |
add_actionspeed_modifier | Add a action speed modifier to a mob. If a variable subtype is passed in as the first argument, it will make a new datum. If ID conflicts, it will overwrite the old ID. |
add_movespeed_modifier | Add a move speed modifier to a mob. If a variable subtype is passed in as the first argument, it will make a new datum. If ID conflicts, it will overwrite the old ID. |
add_to_alive_mob_list | Adds the mob reference to the list of all mobs alive. If mob is cliented, it adds it to the list of all living player-mobs. |
add_to_current_dead_players | Adds the cliented mob reference to either the list of dead player-mobs or to the list of observers, depending on how they joined the game. |
add_to_current_living_antags | Adds the cliented mob reference to the list of living antag player-mobs. |
add_to_current_living_players | Adds the cliented mob reference to the list of living player-mobs. If the mob is an antag, it adds it to the list of living antag player-mobs. |
add_to_dead_mob_list | Adds the mob reference to the list of all the dead mobs. If mob is cliented, it adds it to the list of all dead player-mobs. |
add_to_mob_list | Adds the mob reference to the list and directory of all mobs. Called on Initialize(). |
add_to_player_list | Adds the cliented mob reference to the list of all player-mobs, besides to either the of dead or alive player-mob lists, as appropriate. Called on Login(). |
adjust_blindness | Adjust a mobs blindness by an amount |
adjust_blurriness | Adjust the current blurriness of the mobs vision by amount |
adjust_bodytemperature | Adjust the body temperature of a mob, with min/max settings |
adjust_disgust | Adjust the disgust level of a mob |
adjust_drugginess | Adjust the drugginess of a mob |
adjust_nutrition | Adjust the nutrition of a mob |
adjust_thirst | Adjust the thirst of a mob |
attempt_resist_grab | Proc to resist a grab. moving_resist is TRUE if this began by someone attempting to move. Return FALSE if still grabbed/failed to break out. Use this instead of resist_grab() directly. |
audible_message | Show a message to all mobs in earshot of this one |
blind_eyes | Sets a mob's blindness to an amount if it was not above it already, similar to how status effects work |
blur_eyes | Make the mobs vision blurry |
check_obscured_slots | Checks for slots that are currently obscured by other garments. |
clear_fullscreen | Wipes a fullscreen of a certain category |
clear_typing_indicator | Removes typing indicator. |
create_chat_message | Creates a message overlay at a defined location for a given speaker |
display_typing_indicator | Displays typing indicator. @param timeout_override - Sets how long until this will disappear on its own without the user finishing their message or logging out. Defaults to src.typing_indicator_timeout @param state_override - Sets the state that we will fetch. Defaults to src.get_typing_indicator_icon_state() @param force - shows even if src.typing_indcator_enabled is FALSE. |
dispose_rendering | destroys screen rendering. call on mob del |
dropItemToGround | Used to drop an item (if it exists) to the ground. |
equipped_speed_mods | Gets the combined speed modification of all worn items Except base mob type doesnt really wear items |
examinate | Examine a mob |
generate_typing_indicator | Generates the mutable appearance for typing indicator. Should prevent stuck overlays. |
get_ability_property | Gets an ability property |
get_actionspeed_modifiers | Get the action speed modifiers list of the mob |
get_active_hand | Get the bodypart for whatever hand we have active, Only relevant for carbons |
get_config_multiplicative_speed | Get the global config movespeed of a mob by type |
get_indicator_overlay | Fetches the typing indicator we'll use from GLOB.typing_indicator_overlays |
get_movespeed_modifier_datum | Gets the movespeed modifier datum of a modifier on a mob. Returns null if not found. DANGER: IT IS UP TO THE PERSON USING THIS TO MAKE SURE THE MODIFIER IS NOT MODIFIED IF IT HAPPENS TO BE GLOBAL/CACHED. |
get_movespeed_modifiers | Get the move speed modifiers list of the mob |
get_num_held_items | Find number of held items, multihand compatible |
get_proc_holders | Gets all relevant proc holders for the browser statpenl |
get_spells_for_statpanel | Convert a list of spells into a displyable list for the statpanel |
get_status_tab_items | Adds this list to the output to the stat browser |
get_top_level_mob | SUBTLE COMMAND |
get_typing_indicator_icon_state | Gets the state we will use for typing indicators. Defaults to src.typing_indicator_state |
grant_ability_from_source | Grants an ability from a source |
handle_eye_contact | handle_eye_contact() is called when we examine() something. If we examine an alive mob with a mind who has examined us in the last second within 5 tiles, we make eye contact! |
has_actionspeed_modifier | Is there a actionspeed modifier for this mob |
has_movespeed_modifier | Is there a movespeed modifier for this mob |
hide_fullscreens | Removes fullscreens from client but not the mob |
init_rendering | initializes screen rendering. call on mob new |
initialize_actionspeed | Adds a default action speed |
interact_with | Attempts to open the tgui menu |
list_interaction_attributes | |
onShuttleMove | Mob move procs |
overlay_fullscreen | Adds a fullscreen overlay |
pointed | Point at an atom |
reload_fullscreen | Ensures all fullscreens are on client. |
reload_rendering | loads screen rendering. call on mob login |
remove_ability_from_source | Removes an ability from a source |
remove_actionspeed_modifier | Remove a action speed modifier from a mob, whether static or variable. |
remove_from_alive_mob_list | Removes the mob reference from the list of all mobs alive. If mob is cliented, it removes it from the list of all living player-mobs. |
remove_from_current_dead_players | Removes the mob reference from either the list of dead player-mobs or from the list of observers, depending on how they joined the game. |
remove_from_current_living_antags | Removes the mob reference from the list of living antag player-mobs. |
remove_from_current_living_players | Removes the mob reference from the list of living player-mobs. If the mob is an antag, it removes it from the list of living antag player-mobs. |
remove_from_dead_mob_list | Remvoes the mob reference from list of all the dead mobs. If mob is cliented, it adds it to the list of all dead player-mobs. |
remove_from_mob_list | Removes the mob reference from the list and directory of all mobs. Called on Destroy(). |
remove_from_player_list | Removes the mob reference from the list of all player-mobs, besides from either the of dead or alive player-mob lists, as appropriate. Called on Logout(). |
remove_movespeed_modifier | Remove a move speed modifier from a mob, whether static or variable. |
setGrabState | Updates the grab state of the mob and updates movespeed |
set_ability_property | Sets an ability property |
set_blindness | Force set the blindness of a mob to some level |
set_blurriness | Set the mobs blurriness of vision to an amount |
set_disgust | Set the disgust level of a mob |
set_dizziness | FOrce set the dizzyness of a mob |
set_drugginess | Set the drugginess of a mob |
set_nutrition | Force set the mob nutrition |
set_species | DNA MOB-PROCS |
set_stat | Used to wrap stat setting to trigger on-stat-change functionality. Must be used instead of directly setting a mob's stat var, so that the signal is sent properly. |
shared_ui_interaction | public |
shuttleRotate | Mob rotate procs |
total_multiplicative_slowdown | Calculate the total slowdown of all movespeed modifiers |
update_action_buttons | This proc handles adding all of the mob's actions to their screen |
update_actionspeed | Go through the list of actionspeed modifiers and calculate a final actionspeed. ANY ADD/REMOVE DONE IN UPDATE_actionspeed MUST HAVE THE UPDATE ARGUMENT SET AS FALSE! |
update_blindness | proc that adds and removes blindness overlays when necessary |
update_config_movespeed | Set or update the global movespeed config on a mob |
update_movespeed | Go through the list of movespeed modifiers and calculate a final movespeed. ANY ADD/REMOVE DONE IN UPDATE_MOVESPEED MUST HAVE THE UPDATE ARGUMENT SET AS FALSE! |
visible_message | Adds the functionality to self_message. |
vv_edit_var | Handles the special case of editing the movement var |
wipe_fullscreens | Wipes all fullscreens |
Var Details
LAssailant 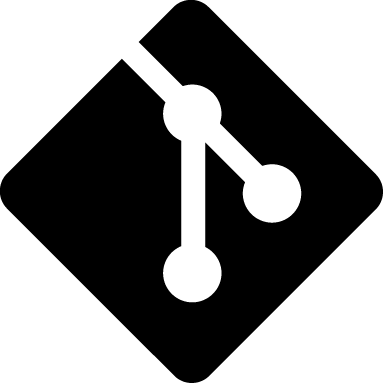
The last mob/living/carbon to push/drag/grab this mob (mostly used by slimes friend recognition)
ability_actions 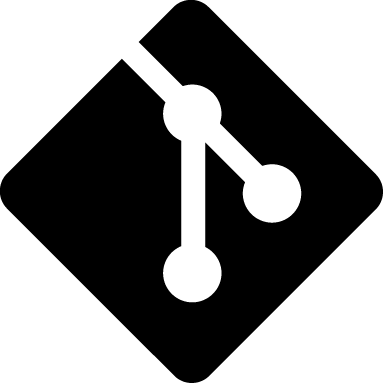
ability = action button instance.
ability_properties 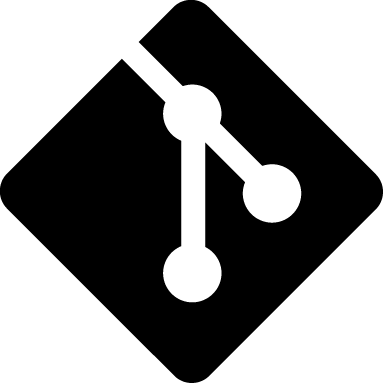
ability = list(data). see __DEFINES/mobs/innate_abilities.dm
action_cooldown_adjust 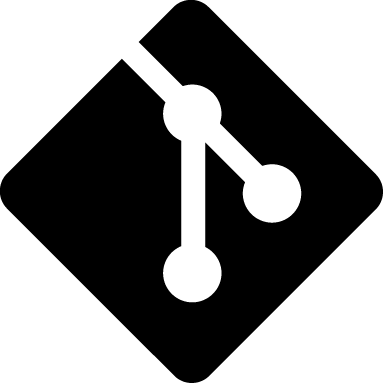
Simple modification variable added to amount on adjust and on checking time since last action using [CheckActionCooldown()]. This should only be manually modified via addition.
action_cooldown_mod 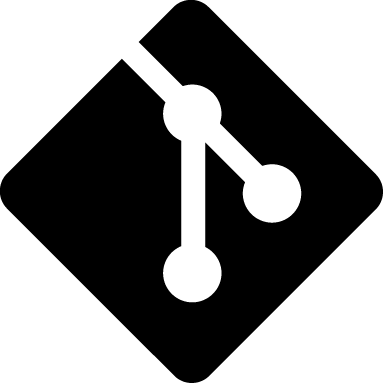
Simple modification variable multiplied to next action modifier on adjust and on checking time since last action using [CheckActionCooldown()]. This should only be manually modified using multipliers.
actionspeed_mod_immunities 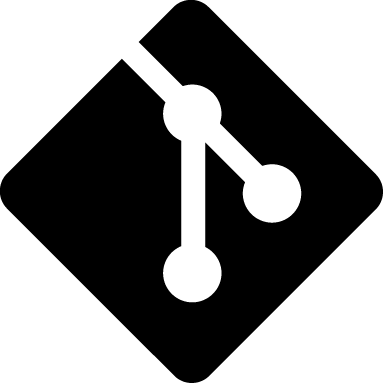
List of action speed modifiers ignored by this mob. List -> List (id) -> List (sources)
actionspeed_modification 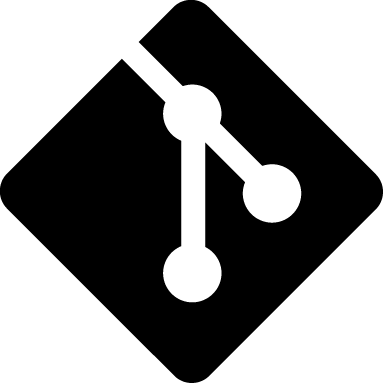
List of action speed modifiers applying to this mob
cached_multiplicative_actions_slowdown 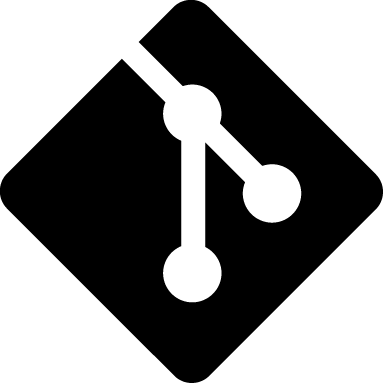
The calculated mob action speed slowdown based on the modifiers list
cached_multiplicative_slowdown 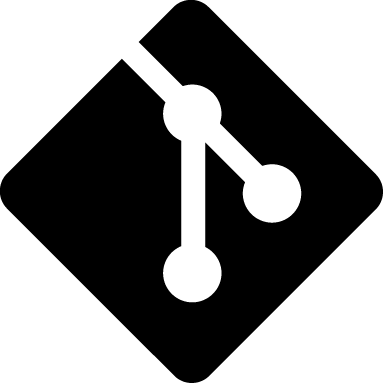
The calculated mob speed slowdown based on the modifiers list
deathsound 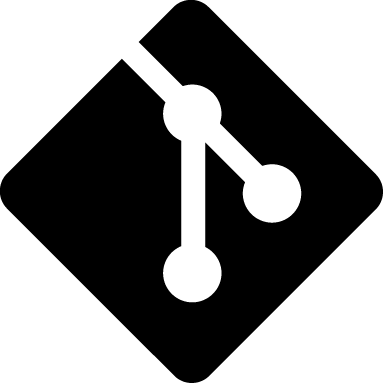
The sound made on death
leave null for no sound. used for *deathgasp
do_afters 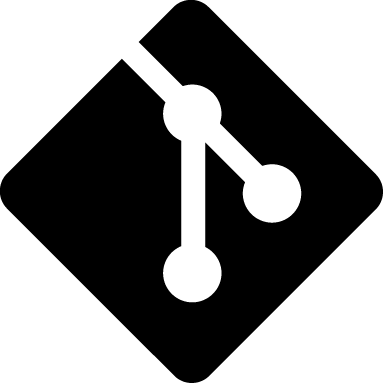
For storing what do_after's someone has, in case we want to restrict them to only one of a certain do_after at a time
examine_cursor_icon 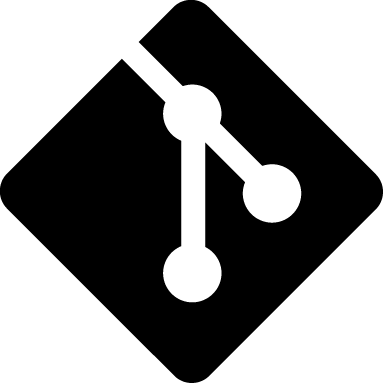
Cursor icon used when holding shift over things
focus 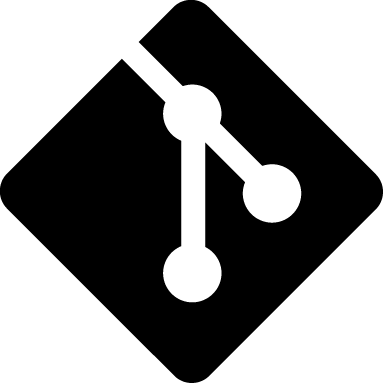
What receives our keyboard input. src by default.
fullscreens 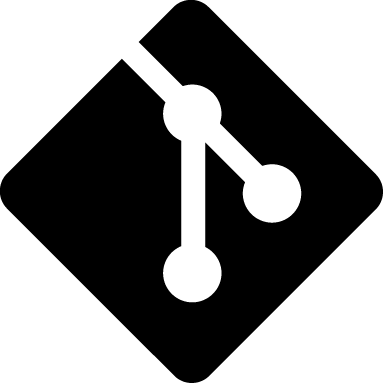
Fullscreen objects
innate_abilities 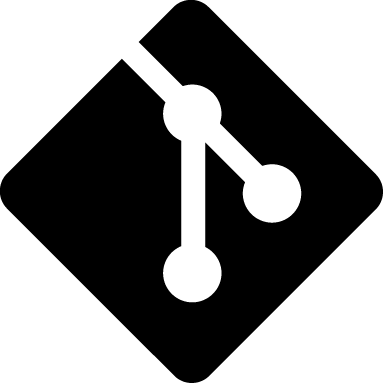
Ability system based on action buttons. Can be ported to base /mob or /mob/living later if needed, easily - the procs are currently on living/carbon/human/innate_abilities.dm datum traits-style lazylist of abilities
is_shifted 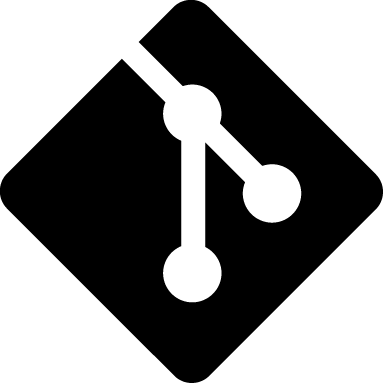
Whether the mob is pixel shifted or not
last_action 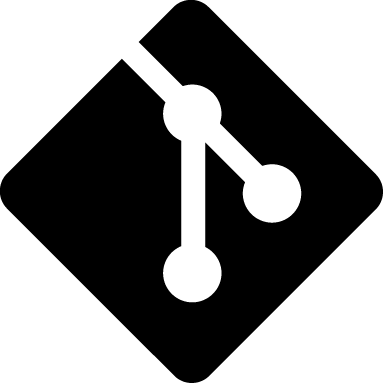
Generic clickdelay variable. Marks down the last world.time we did something that should cause or impact generic clickdelay. This should be directly set or set using [DelayNextAction()]. This should only be checked using [CheckActionCooldown()].
last_action_immediate 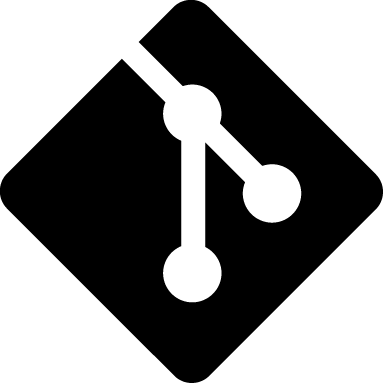
The difference between the above and this is this is set immediately before even the pre-attack begins to ensure clickdelay is respected. Then, it is flushed or discarded using [FlushLastAttack()] or [DiscardLastAttack()] respectively.
mob_transforming 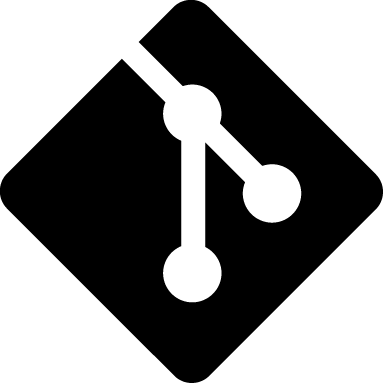
Whether or not the mob is currently being transformed into another mob or into another state of being. This will prevent it from moving or doing realistically anything. Don't you DARE use this for a cheap way to ensure someone is stunned in your code.
mock_client 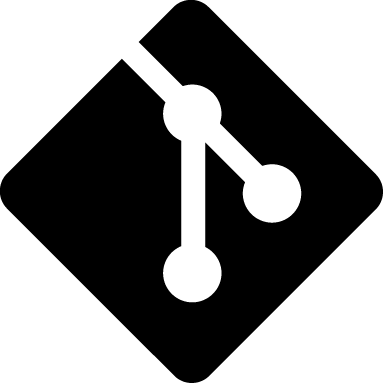
A mock client, provided by tests and friends
movespeed_mod_immunities 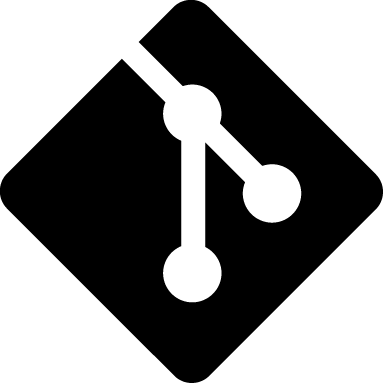
List of movement speed modifiers ignored by this mob. List -> List (id) -> List (sources)
movespeed_modification 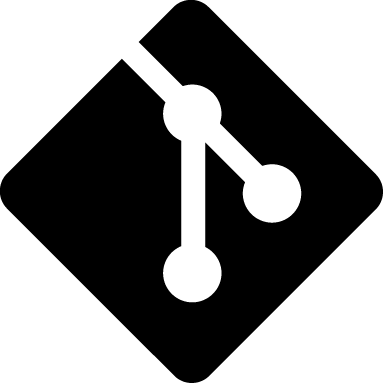
List of movement speed modifiers applying to this mob
next_action 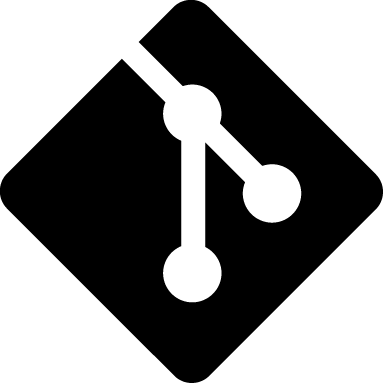
Generic clickdelay variable. Next world.time we should be able to do something that respects generic clickdelay. This should be set using [DelayNextAction()] This should only be checked using [CheckActionCooldown()].
next_action_immediate 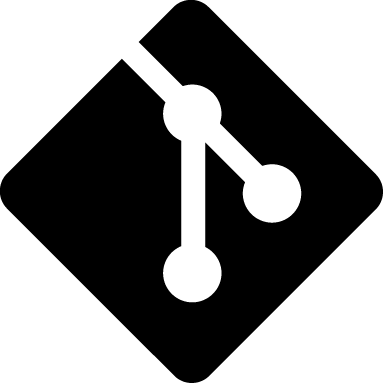
Ditto
next_resist 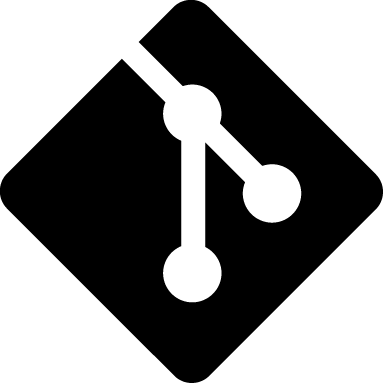
Minimum world time for another resist. This should only be checked using [CheckResistCooldown()].
passthroughable 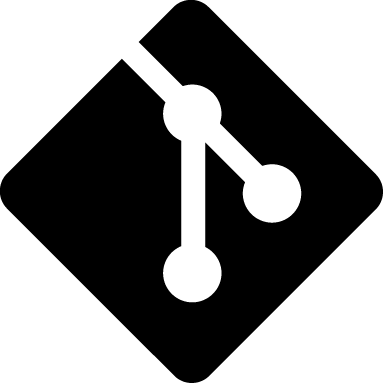
Takes the four cardinal direction defines. Any atoms moving into this atom's tile will be allowed to from the added directions.
resist_cooldown 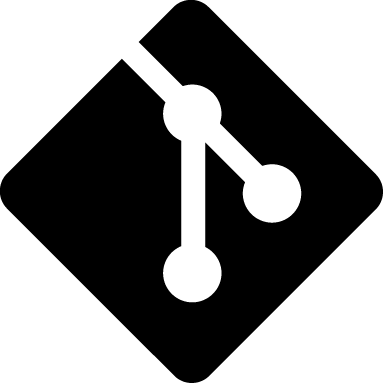
How long we should wait before allowing another resist. This should only be manually modified using multipliers.
shifting 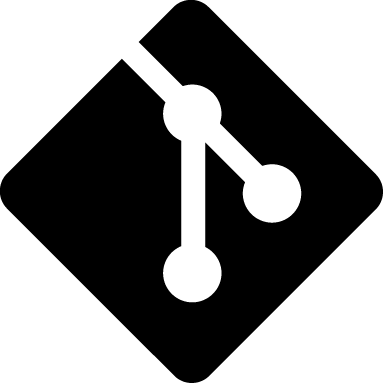
If we are in the shifting setting.
sound_environment_override 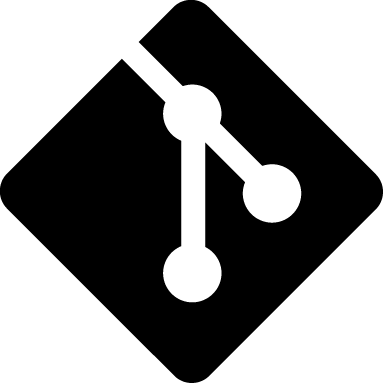
Override for sound_environments. If this is set the user will always hear a specific type of reverb (Instead of the area defined reverb)
tgui_open_uis 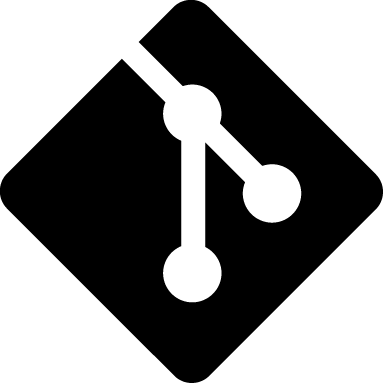
global
Tracks open UIs for a user.
typing_indicator_current 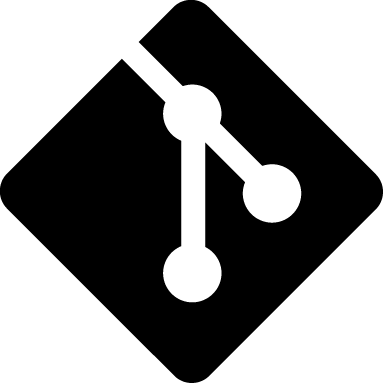
Current state of our typing indicator. Used for cut overlay, DO NOT RUNTIME ASSIGN OTHER THAN FROM SHOW/CLEAR. Used to absolutely ensure we do not get stuck overlays.
typing_indicator_enabled 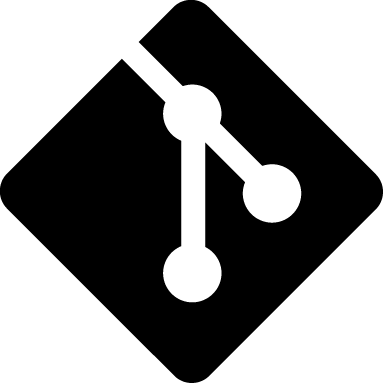
////TYPING INDICATORS/////// Set to true if we want to show typing indicators.
typing_indicator_state 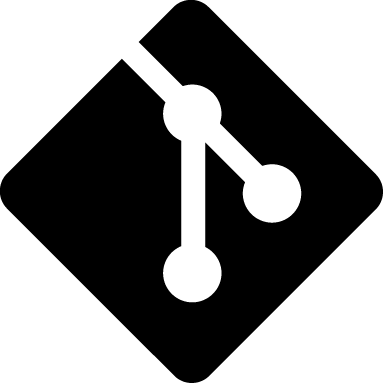
Default icon_state of our typing indicator. Currently only supports paths (because anything else is, as of time of typing this, unnecesary.
typing_indicator_timerid 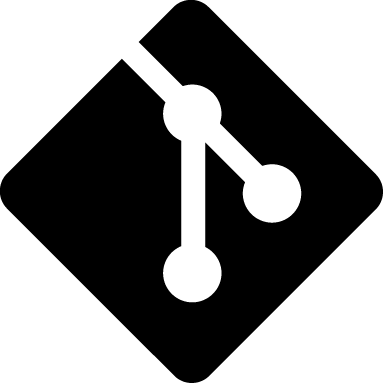
The timer that will remove our indicator for early aborts (like when an user finishes their message)
unarmed_attack_speed 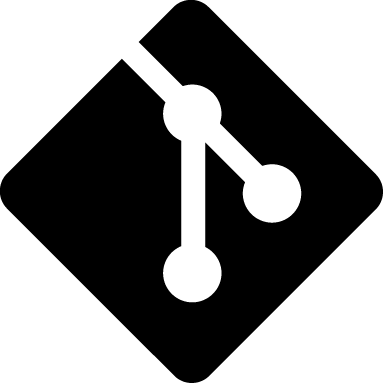
Default clickdelay for an UnarmedAttack() that successfully passes. Respects action_cooldown_mod.
Proc Details
CheckActionCooldown
Checks if we can do another action. Returns TRUE if we can and FALSE if we cannot.
@params
- cooldown - Time required since last action. Defaults to 0.5
- from_next_action - Defaults to FALSE. Should we check from the tail end of next_action instead of last_action?
- ignore_mod - Defaults to FALSE. Ignore all adjusts and multipliers. Do not use this unless you know what you are doing and have a good reason.
- ignore_next_action - Defaults to FALSE. Ignore next_action and only care about cooldown param and everything else. Generally unused.
- immediate - Defaults to FALSE. Checks last action using immediate, used on the head end of an attack. This is to prevent colliding attacks in case of sleep. Not that you should sleep() in an attack but.. y'know.
CheckResistCooldown
Checks if we can resist again.
CommonClickOn
Common mob click code
DelayNextAction
Applies a delay to next_action before we can do our next action.
@params
- amount - Amount to delay by
- ignore_mod - ignores next action adjust and mult
- considered_action - Defaults to TRUE - If TRUE, sets last_action to world.time.
- immediate - defaults to TRUE - if TRUE, writes to cached/last_attack_immediate instead of last_attack. This ensures it can't collide with any delay checks in the actual attack.
- flush - defaults to FALSE - Use this while using this proc outside of clickcode to ensure everything is set properly. This should never be set to TRUE if this is called from clickcode.
DiscardCurrentAction
Discards last_action and next_action
Dizzy
Set the dizzyness of a mob to a passed in amount
Except if dizziness is already higher in which case it does nothing
EstimatedNextActionTime
Get estimated time of next attack.
FlushCurrentAction
Flushes last_action and next_action
GetActionCooldownAdjust
Gets action_cooldown_adjust
GetActionCooldownMod
Gets action_cooldown_mod.
Jitter
Set the jitter of a mob
MarkResistTime
Mark the last resist as now.
@params
- extra_cooldown - Extra cooldown to apply to next_resist. Defaults to this mob's resist_cooldown.
- override - Defaults to FALSE - if TRUE, extra_cooldown will replace the old next_resist even if the old is longer.
SetNextAction
Sets our next action to. The difference is DelayNextAction cannot reduce next_action under any circumstances while this can.
add_actionspeed_modifier
Add a action speed modifier to a mob. If a variable subtype is passed in as the first argument, it will make a new datum. If ID conflicts, it will overwrite the old ID.
add_movespeed_modifier
Add a move speed modifier to a mob. If a variable subtype is passed in as the first argument, it will make a new datum. If ID conflicts, it will overwrite the old ID.
add_to_alive_mob_list
Adds the mob reference to the list of all mobs alive. If mob is cliented, it adds it to the list of all living player-mobs.
add_to_current_dead_players
Adds the cliented mob reference to either the list of dead player-mobs or to the list of observers, depending on how they joined the game.
add_to_current_living_antags
Adds the cliented mob reference to the list of living antag player-mobs.
add_to_current_living_players
Adds the cliented mob reference to the list of living player-mobs. If the mob is an antag, it adds it to the list of living antag player-mobs.
add_to_dead_mob_list
Adds the mob reference to the list of all the dead mobs. If mob is cliented, it adds it to the list of all dead player-mobs.
add_to_mob_list
Adds the mob reference to the list and directory of all mobs. Called on Initialize().
add_to_player_list
Adds the cliented mob reference to the list of all player-mobs, besides to either the of dead or alive player-mob lists, as appropriate. Called on Login().
adjust_blindness
Adjust a mobs blindness by an amount
Will apply the blind alerts if needed
adjust_blurriness
Adjust the current blurriness of the mobs vision by amount
adjust_bodytemperature
Adjust the body temperature of a mob, with min/max settings
adjust_disgust
Adjust the disgust level of a mob
adjust_drugginess
Adjust the drugginess of a mob
adjust_nutrition
Adjust the nutrition of a mob
adjust_thirst
Adjust the thirst of a mob
attempt_resist_grab
Proc to resist a grab. moving_resist is TRUE if this began by someone attempting to move. Return FALSE if still grabbed/failed to break out. Use this instead of resist_grab() directly.
audible_message
Show a message to all mobs in earshot of this one
This would be for audible actions by the src mob
vars:
- message is the message output to anyone who can hear.
- self_message (optional) is what the src mob hears.
- deaf_message (optional) is what deaf people will see.
- hearing_distance (optional) is the range, how many tiles away the message can be heard.
- ignored_mobs (optional) doesn't show any message to any given mob in the list.
blind_eyes
Sets a mob's blindness to an amount if it was not above it already, similar to how status effects work
blur_eyes
Make the mobs vision blurry
check_obscured_slots
Checks for slots that are currently obscured by other garments.
clear_fullscreen
Wipes a fullscreen of a certain category
Second argument is for animation delay.
clear_typing_indicator
Removes typing indicator.
create_chat_message
Creates a message overlay at a defined location for a given speaker
Arguments:
- speaker - The atom who is saying this message
- message_language - The language that the message is said in
- raw_message - The text content of the message
- spans - Additional classes to be added to the message
- message_mode - Bitflags relating to the mode of the message
display_typing_indicator
Displays typing indicator. @param timeout_override - Sets how long until this will disappear on its own without the user finishing their message or logging out. Defaults to src.typing_indicator_timeout @param state_override - Sets the state that we will fetch. Defaults to src.get_typing_indicator_icon_state() @param force - shows even if src.typing_indcator_enabled is FALSE.
dispose_rendering
destroys screen rendering. call on mob del
dropItemToGround
Used to drop an item (if it exists) to the ground.
- Will pass as TRUE is successfully dropped, or if there is no item to drop.
- Will pass FALSE if the item can not be dropped due to TRAIT_NODROP via doUnEquip() If the item can be dropped, it will be forceMove()'d to the ground and the turf's Entered() will be called.
equipped_speed_mods
Gets the combined speed modification of all worn items Except base mob type doesnt really wear items
examinate
Examine a mob
mob verbs are faster than object verbs. See this byond forum post for why this isn't atom/verb/examine()
generate_typing_indicator
Generates the mutable appearance for typing indicator. Should prevent stuck overlays.
get_ability_property
Gets an ability property
get_actionspeed_modifiers
Get the action speed modifiers list of the mob
get_active_hand
Get the bodypart for whatever hand we have active, Only relevant for carbons
get_config_multiplicative_speed
Get the global config movespeed of a mob by type
get_indicator_overlay
Fetches the typing indicator we'll use from GLOB.typing_indicator_overlays
get_movespeed_modifier_datum
Gets the movespeed modifier datum of a modifier on a mob. Returns null if not found. DANGER: IT IS UP TO THE PERSON USING THIS TO MAKE SURE THE MODIFIER IS NOT MODIFIED IF IT HAPPENS TO BE GLOBAL/CACHED.
get_movespeed_modifiers
Get the move speed modifiers list of the mob
get_num_held_items
Find number of held items, multihand compatible
get_proc_holders
Gets all relevant proc holders for the browser statpenl
get_spells_for_statpanel
Convert a list of spells into a displyable list for the statpanel
Shows charge and other important info
get_status_tab_items
Adds this list to the output to the stat browser
get_top_level_mob
SUBTLE COMMAND
get_typing_indicator_icon_state
Gets the state we will use for typing indicators. Defaults to src.typing_indicator_state
grant_ability_from_source
Grants an ability from a source
handle_eye_contact
handle_eye_contact() is called when we examine() something. If we examine an alive mob with a mind who has examined us in the last second within 5 tiles, we make eye contact!
Note that if either party has their face obscured, the other won't get the notice about the eye contact Also note that examine_more() doesn't proc this or extend the timer, just because it's simpler this way and doesn't lose much. The nice part about relying on examining is that we don't bother checking visibility, because we already know they were both visible to each other within the last second, and the one who triggers it is currently seeing them
has_actionspeed_modifier
Is there a actionspeed modifier for this mob
has_movespeed_modifier
Is there a movespeed modifier for this mob
hide_fullscreens
Removes fullscreens from client but not the mob
init_rendering
initializes screen rendering. call on mob new
initialize_actionspeed
Adds a default action speed
interact_with
Attempts to open the tgui menu
list_interaction_attributes
# Interactions code by HONKERTRON feat TestUnit
- Contains a lot ammount of ERP and MEHANOYEBLYA
- CREDIT TO ATMTA STATION FOR MOST OF THIS CODE, I ONLY MADE IT WORK IN /vg/ - Matt
- Rewritten 30/08/16 by Zuhayr, sry if I removed anything important.
- I removed ERP and replaced it with handholding. Nothing of worth was lost. - Vic
- Fuck you, Vic. ERP is back. - TT
-
using var/ on everything, also TRUE
- "TGUIzes" the panel because yes - SandPoot
- Makes all the code good because yes as well - SandPoot
onShuttleMove
Mob move procs
overlay_fullscreen
Adds a fullscreen overlay
@params
- category - string - must exist. will overwrite any other screen in this category. defaults to type.
- type - the typepath of the screen
- severity - severity - different screen objects have differing severities
pointed
Point at an atom
mob verbs are faster than object verbs. See this byond forum post for why this isn't atom/verb/pointed()
note: ghosts can point, this is intended
visible_message will handle invisibility properly
overridden here and in /mob/dead/observer for different point span classes and sanity checks
reload_fullscreen
Ensures all fullscreens are on client.
reload_rendering
loads screen rendering. call on mob login
remove_ability_from_source
Removes an ability from a source
remove_actionspeed_modifier
Remove a action speed modifier from a mob, whether static or variable.
remove_from_alive_mob_list
Removes the mob reference from the list of all mobs alive. If mob is cliented, it removes it from the list of all living player-mobs.
remove_from_current_dead_players
Removes the mob reference from either the list of dead player-mobs or from the list of observers, depending on how they joined the game.
remove_from_current_living_antags
Removes the mob reference from the list of living antag player-mobs.
remove_from_current_living_players
Removes the mob reference from the list of living player-mobs. If the mob is an antag, it removes it from the list of living antag player-mobs.
remove_from_dead_mob_list
Remvoes the mob reference from list of all the dead mobs. If mob is cliented, it adds it to the list of all dead player-mobs.
remove_from_mob_list
Removes the mob reference from the list and directory of all mobs. Called on Destroy().
remove_from_player_list
Removes the mob reference from the list of all player-mobs, besides from either the of dead or alive player-mob lists, as appropriate. Called on Logout().
remove_movespeed_modifier
Remove a move speed modifier from a mob, whether static or variable.
setGrabState
Updates the grab state of the mob and updates movespeed
set_ability_property
Sets an ability property
set_blindness
Force set the blindness of a mob to some level
set_blurriness
Set the mobs blurriness of vision to an amount
set_disgust
Set the disgust level of a mob
set_dizziness
FOrce set the dizzyness of a mob
set_drugginess
Set the drugginess of a mob
set_nutrition
Force set the mob nutrition
set_species
DNA MOB-PROCS
set_stat
Used to wrap stat setting to trigger on-stat-change functionality. Must be used instead of directly setting a mob's stat var, so that the signal is sent properly.
shared_ui_interaction
public
Standard interaction/sanity checks. Different mob types may have overrides.
return UI_state The state of the UI.
shuttleRotate
Mob rotate procs
total_multiplicative_slowdown
Calculate the total slowdown of all movespeed modifiers
update_action_buttons
This proc handles adding all of the mob's actions to their screen
If you just need to update existing buttons, use [/mob/proc/update_mob_action_buttons]!
Arguments:
- update_flags - reload_screen - bool, if TRUE, this proc will add the button to the screen of the passed mob as well
update_actionspeed
Go through the list of actionspeed modifiers and calculate a final actionspeed. ANY ADD/REMOVE DONE IN UPDATE_actionspeed MUST HAVE THE UPDATE ARGUMENT SET AS FALSE!
update_blindness
proc that adds and removes blindness overlays when necessary
update_config_movespeed
Set or update the global movespeed config on a mob
update_movespeed
Go through the list of movespeed modifiers and calculate a final movespeed. ANY ADD/REMOVE DONE IN UPDATE_MOVESPEED MUST HAVE THE UPDATE ARGUMENT SET AS FALSE!
visible_message
Adds the functionality to self_message.
vv_edit_var
Handles the special case of editing the movement var
wipe_fullscreens
Wipes all fullscreens